Form Builder - Fields
Radio
Overview
The radio input provides a radio button group for selecting a single value from a list of predefined options:
use Filament\Forms\Components\Radio; Radio::make('status') ->options([ 'draft' => 'Draft', 'scheduled' => 'Scheduled', 'published' => 'Published' ])
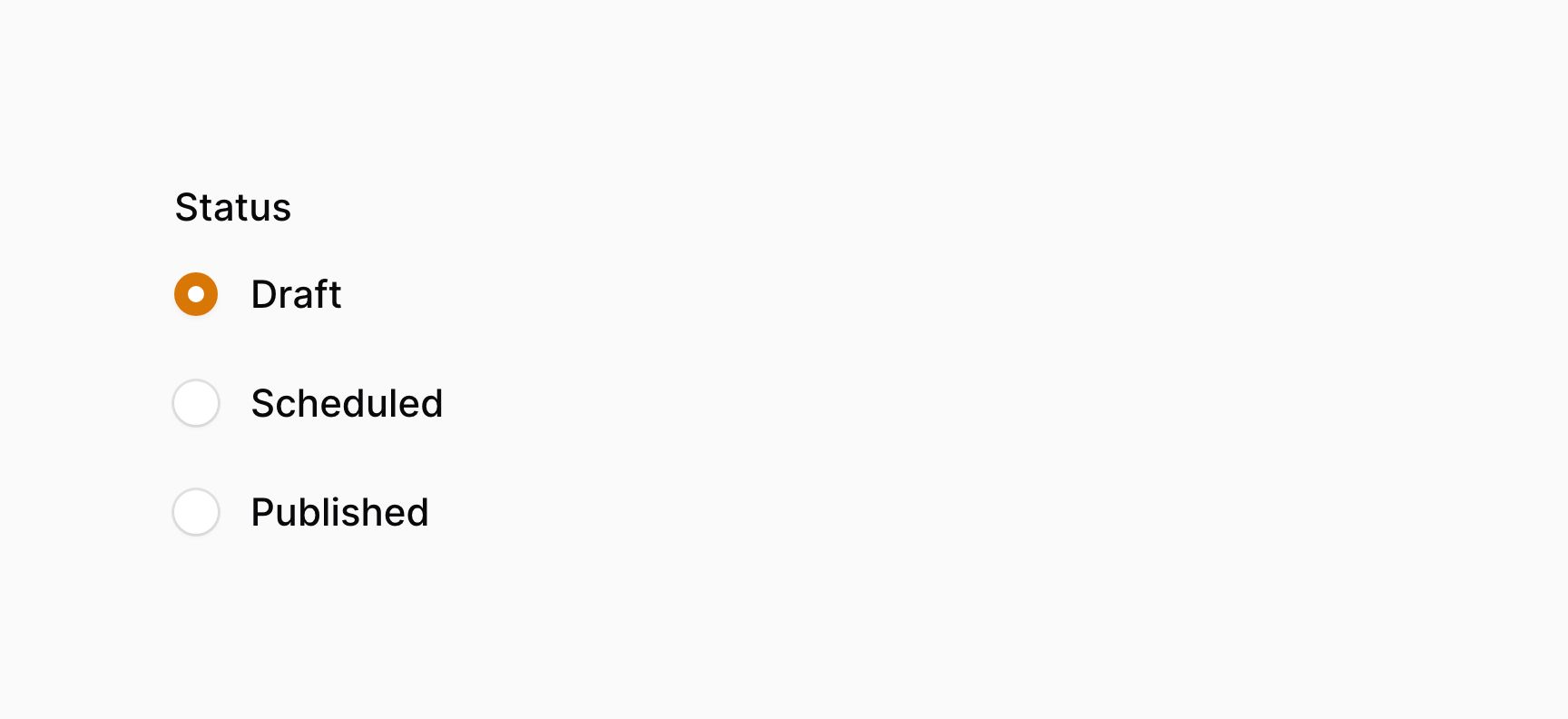
Setting option descriptions
You can optionally provide descriptions to each option using the descriptions()
method:
use Filament\Forms\Components\Radio; Radio::make('status') ->options([ 'draft' => 'Draft', 'scheduled' => 'Scheduled', 'published' => 'Published' ]) ->descriptions([ 'draft' => 'Is not visible.', 'scheduled' => 'Will be visible.', 'published' => 'Is visible.' ])
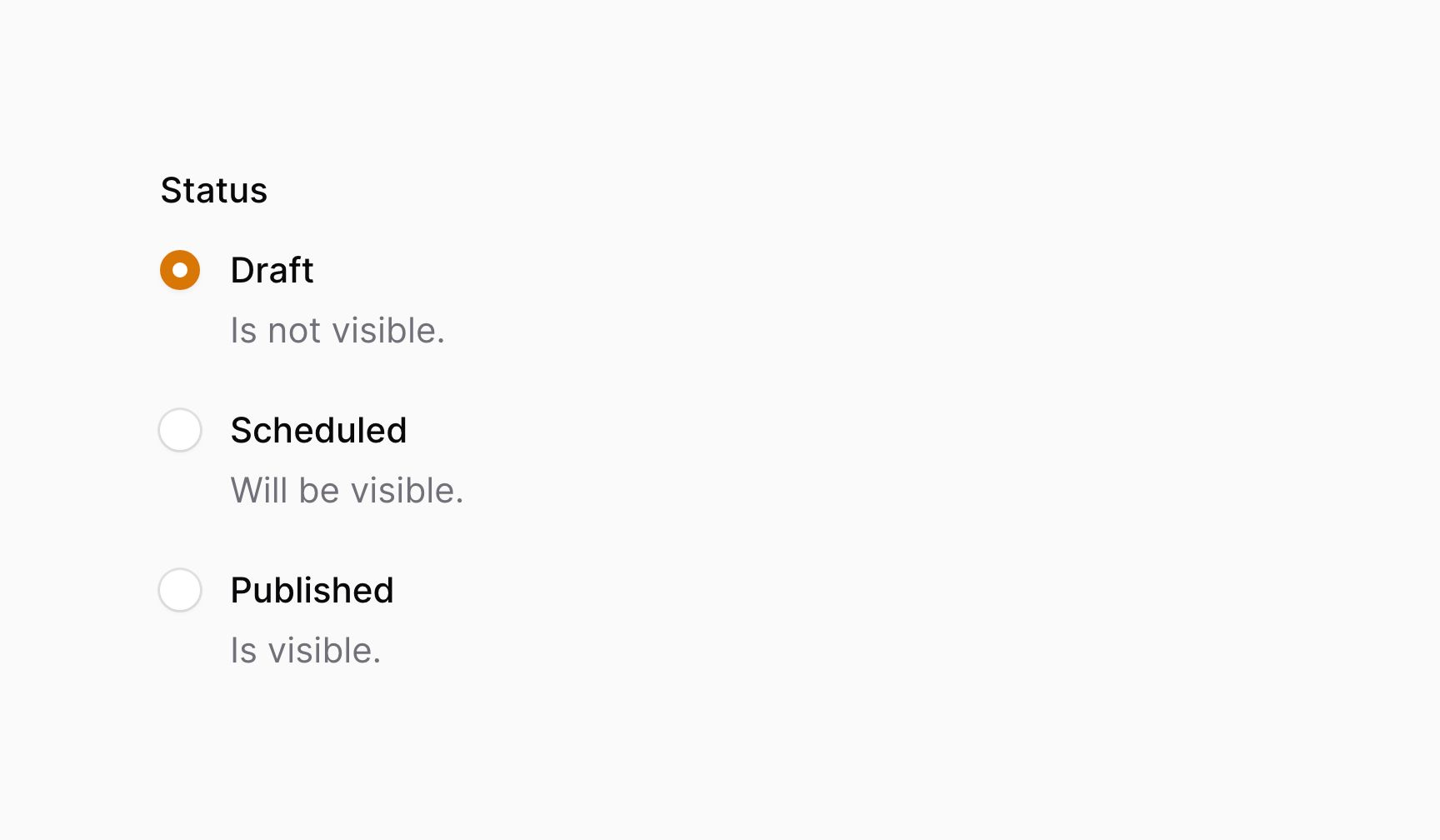
Be sure to use the same key
in the descriptions array as the key
in the option array so the right description matches the right option.
Boolean options
If you want a simple boolean radio button group, with "Yes" and "No" options, you can use the boolean()
method:
Radio::make('feedback') ->label('Like this post?') ->boolean()

Positioning the options inline with the label
You may wish to display the options inline()
with the label instead of below it:
Radio::make('feedback') ->label('Like this post?') ->boolean() ->inline()

Positioning the options inline with each other but below the label
You may wish to display the options inline()
with each other but below the label:
Radio::make('feedback') ->label('Like this post?') ->boolean() ->inline() ->inlineLabel(false)
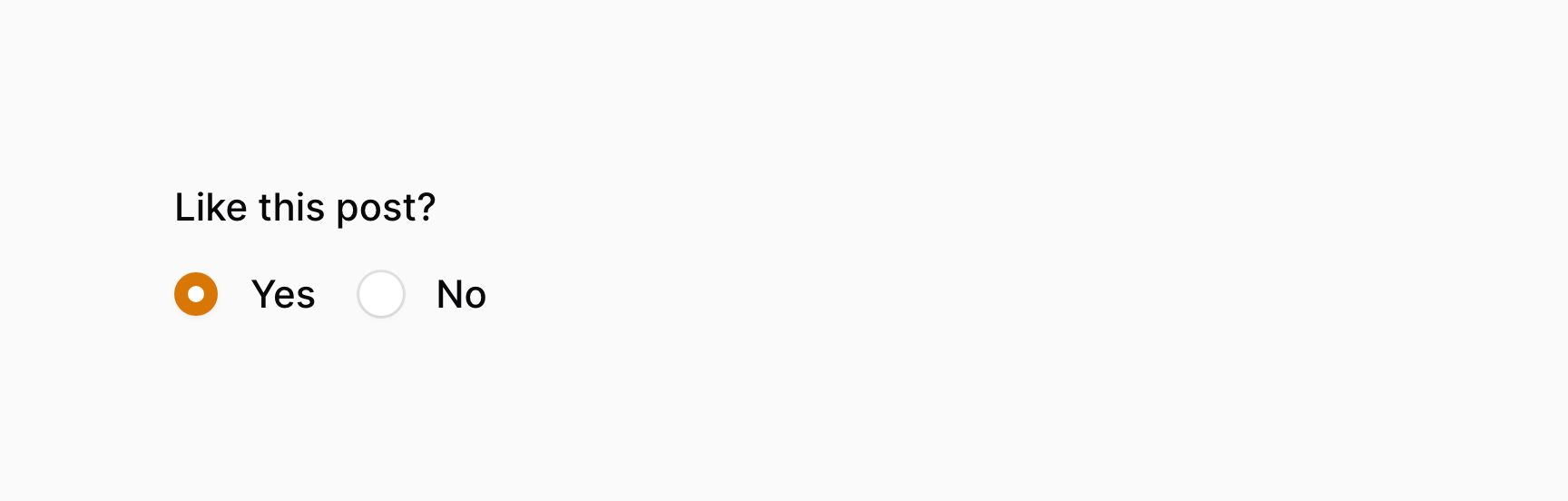
Disabling specific options
You can disable specific options using the disableOptionWhen()
method. It accepts a closure, in which you can check if the option with a specific $value
should be disabled:
use Filament\Forms\Components\Radio; Radio::make('status') ->options([ 'draft' => 'Draft', 'scheduled' => 'Scheduled', 'published' => 'Published', ]) ->disableOptionWhen(fn (string $value): bool => $value === 'published')

If you want to retrieve the options that have not been disabled, e.g. for validation purposes, you can do so using getEnabledOptions()
:
use Filament\Forms\Components\Radio; Radio::make('status') ->options([ 'draft' => 'Draft', 'scheduled' => 'Scheduled', 'published' => 'Published', ]) ->disableOptionWhen(fn (string $value): bool => $value === 'published') ->in(fn (Radio $component): array => array_keys($component->getEnabledOptions()))
Edit on GitHubStill need help? Join our Discord community or open a GitHub discussion