表单构造器
开始
概述
Filament 表单构造器允许你在应用中轻松创建动态表单。使用表单构造器,你可以将表单添加到 Livewire 组件。此外,它可以在其他 Filament 包使用,在应用资源、Action 模态框、表格过滤器等中渲染表单。了解如何创建表单本质就是学习如何使用这些 Filament 包。
本教程将带你一起了解通过 Filament 表单构造器创建表单的基础。如果你计划将新表单添加到自己的 Livewire 组件,你可以先添加完表单,再回来。如果你要将表单添加到应用资源或者其他 Filament 包中,可以开始了!
表单 schema
所有的 Filament 表单都有 "schema"。这是一个数组,其中包含表单字段和布局组件。
表单字段是用户用来填写数据的输入框。比如,HTML 的 <input>
或者 <select>
元素。每个字段都有它们自己的 PHP 类。比如,TextInput
类用于渲染文本输入框字段,Select
类用于渲染下拉列表字段。你可在此处查看可用字段完整清单。
布局组件用于分组字段,并控制他们的展示方式。比如,你可以使用 Grid
组件去并排展示多个字段,或者使用 Wizard
将不同字段分离到多步骤表单中。你可以深度嵌套布局组件,以创建复杂的响应性 UI。你可以在此处查看可用布局组件完整清单。
添加字段到表单 schema
使用 make()
方法初始化字段或布局组件,并使用多字段创建 schema 数组:
use Filament\Forms\Components\RichEditor;use Filament\Forms\Components\TextInput;use Filament\Forms\Form; public function form(Form $form): Form{ return $form ->schema([ TextInput::make('title'), TextInput::make('slug'), RichEditor::make('content'), ]);}

面板或者其他包中的表单通常默认有两列。对于自定义表单,你可以使用 columns()
方法,完成相同的效果:
$form ->schema([ // ... ]) ->columns(2);
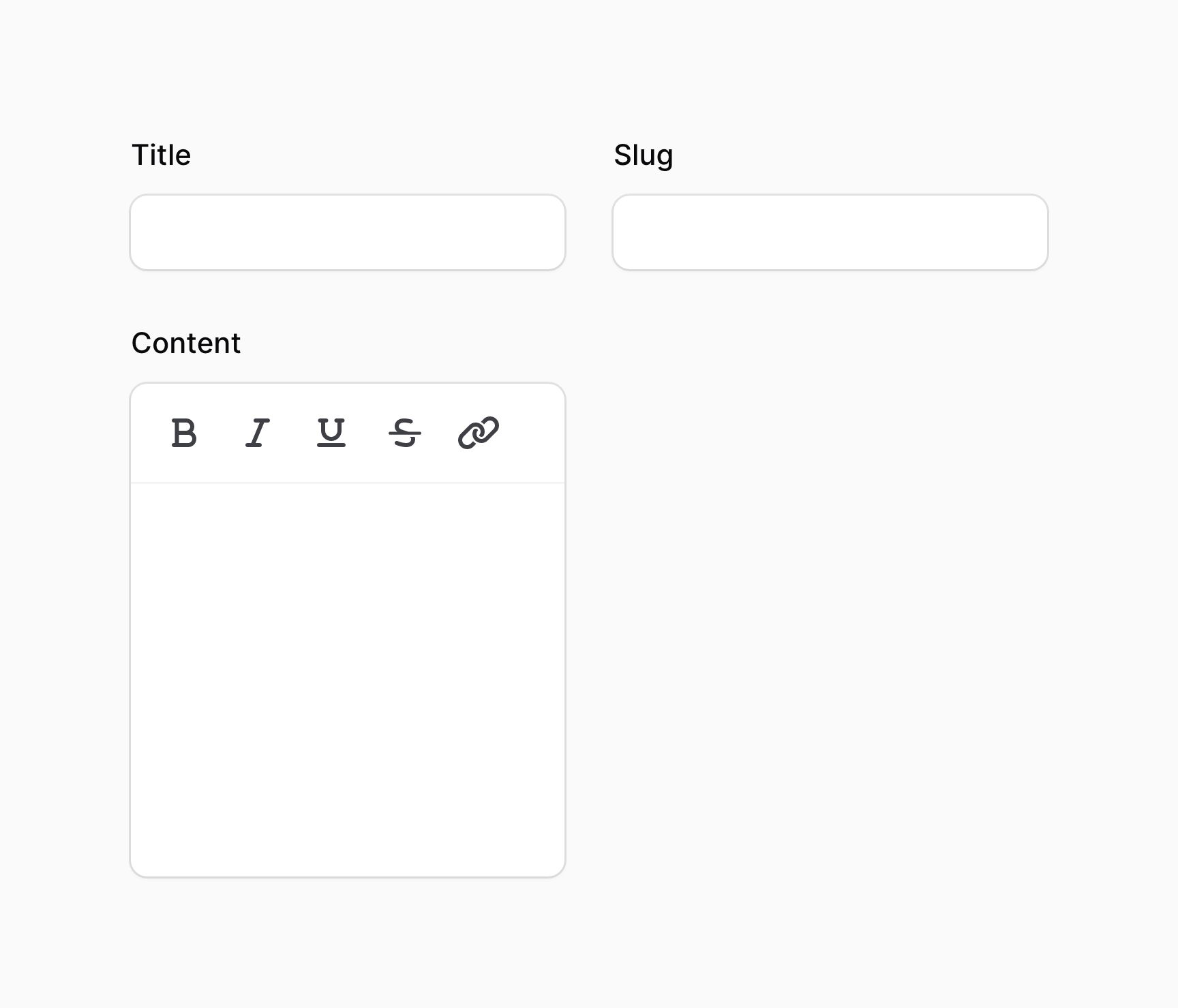
现在,RichEditor
只消费可用宽度的一半。我们可以使用 columnSpan()
方法使之占用整个宽度:
use Filament\Forms\Components\RichEditor;use Filament\Forms\Components\TextInput; [ TextInput::make('title'), TextInput::make('slug'), RichEditor::make('content') ->columnSpan(2), // or `columnSpan('full')`]
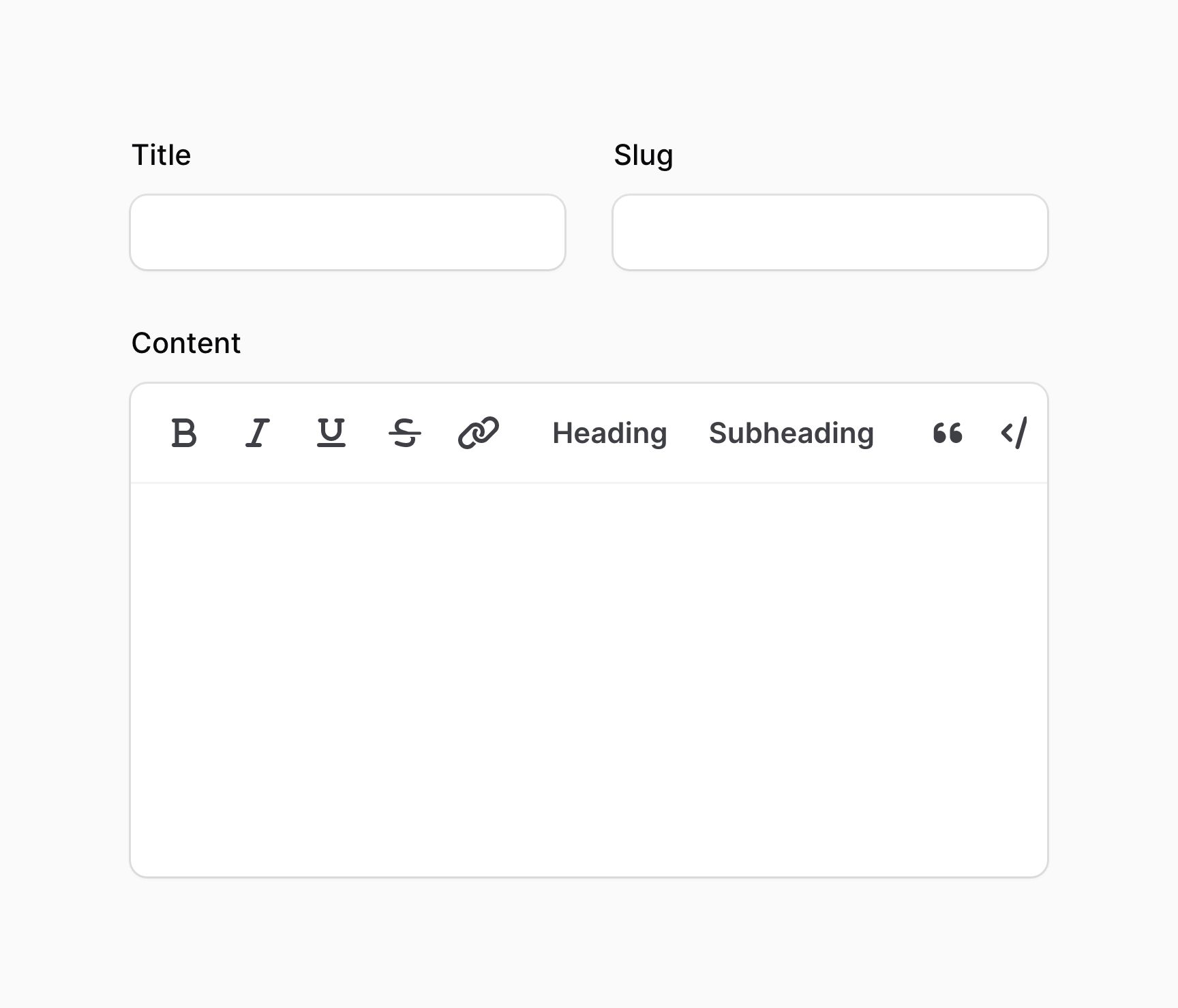
你可以在布局文档中了解更多列和跨度的信息。甚至可以使之以响应式呈现。
添加布局组件到表单 schema
让我们在表单中添加一个新的 Section
。Section
是布局组件,它允许你为一组字段添加标题和描述。也允许内部折叠,用以在长表单中节省空间。
use Filament\Forms\Components\RichEditor;use Filament\Forms\Components\Section;use Filament\Forms\Components\TextInput; [ TextInput::make('title'), TextInput::make('slug'), RichEditor::make('content') ->columnSpan(2), Section::make('Publishing') ->description('Settings for publishing this post.') ->schema([ // ... ]),]
本例中,你可以看到 Section
组件有它自己的 schema()
方法。你可以在它内部嵌套其他字段及布局组件:
use Filament\Forms\Components\DateTimePicker;use Filament\Forms\Components\Section;use Filament\Forms\Components\Select; Section::make('Publishing') ->description('Settings for publishing this post.') ->schema([ Select::make('status') ->options([ 'draft' => 'Draft', 'reviewing' => 'Reviewing', 'published' => 'Published', ]), DateTimePicker::make('published_at'), ])
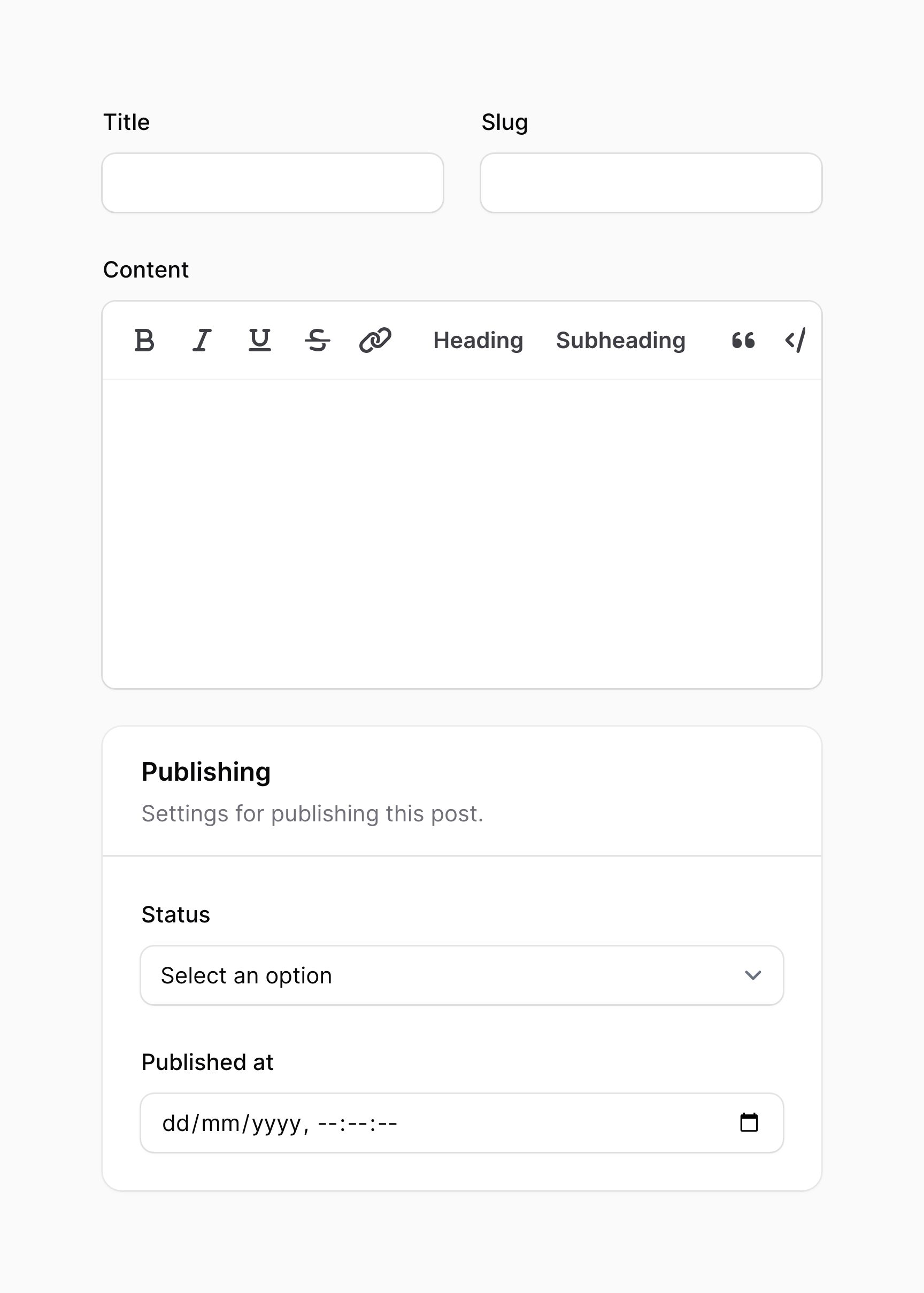
该分区(section)现在包含一个 Select
字段和一个 DateTimePicker
字段。你可以在相应文档页面中了解这些字段以及他们的功能。
验证字段
在 Laravel 中,验证规则通常定义在类似于 ['required', 'max:255']
这样的数组中,或者 required|max:255
这样的连接字符串中。如果你只处理后端的简单表单请求,这是可以的。不过 Filament 也可以提供用户前端验证,这样他们可以在发起后端请求前,修正他们的错误。
在 Filament 中,你可以使用注入 required()
和 maxLength()
这样的方法,将验证规则添加到字段中。该方式也优于 Laravel 的验证验证语法,因为 IDE 可以自动补全这些方法:
use Filament\Forms\Components\DateTimePicker;use Filament\Forms\Components\RichEditor;use Filament\Forms\Components\Section;use Filament\Forms\Components\Select;use Filament\Forms\Components\TextInput; [ TextInput::make('title') ->required() ->maxLength(255), TextInput::make('slug') ->required() ->maxLength(255), RichEditor::make('content') ->columnSpan(2) ->maxLength(65535), Section::make('Publishing') ->description('Settings for publishing this post.') ->schema([ Select::make('status') ->options([ 'draft' => 'Draft', 'reviewing' => 'Reviewing', 'published' => 'Published', ]) ->required(), DateTimePicker::make('published_at'), ]),]
本例中,有些字段是必须的 required
,有些有 maxLength()
限制。我们有大部分 Laravel 验证规则对应的方法,甚至你可以添加自己的自定义规则。
依赖字段
由于所有 Filament 表单是基于 Livewire 创建的,表单 Schema 是完全动态的。其中有许多可能,此处是一些如何使用以对你有利的例子:
字段可以依据其他字段的值隐藏或者显示。上面的表单中,我们可以 published_at
时间戳字段,直至 status
字段设置成 published
。将闭包传递到 hidden()
方法,可以实现该功能;这将允许你在使用表单时动态隐藏或展示字段。闭包可以访问许多有用的参数,比如 $get
,你可以在此处查看所有参数清单。你所依赖的字段(本例中的 status
)需要设置成 live()
,用来告知表单在每次修改时重载 schema。
use Filament\Forms\Components\DateTimePicker;use Filament\Forms\Components\Select;use Filament\Forms\Get; [ Select::make('status') ->options([ 'draft' => 'Draft', 'reviewing' => 'Reviewing', 'published' => 'Published', ]) ->required() ->live(), DateTimePicker::make('published_at') ->hidden(fn (Get $get) => $get('status') !== 'published'),]
不只是 hidden()
,所有的 Filament 表单方法都支持像这样的闭包。你可以用它来基于其他字段修改标签、占位符,甚至于字段的选项。你甚至可以用它来为表单添加新字段或者删除字段。这是一个你可以用最小的努力来创建复杂表单的强大工具。
字段也可以添加数据到其他字段。比如你可以设置标题,使之修改时自动生成 slug。该功能可以通过传递闭包到 afterStateUpdated()
方法实现,该方法会在每次标题改变时运行。该闭包可以方法标题($state
)以及一个用以设置 slug 字段值的函数($set
)。你可以到此处查看闭包参数完整清单。你所有依赖的字段(此处的 title
)需要设置为 live()
,用来告知表单在每次修改时重载并设置 slug。
use Filament\Forms\Components\TextInput;use Filament\Forms\Set;use Illuminate\Support\Str; [ TextInput::make('title') ->required() ->maxLength(255) ->live() ->afterStateUpdated(function (Set $set, $state) { $set('slug', Str::slug($state)); }), TextInput::make('slug') ->required() ->maxLength(255),]
下一步
你已经阅读完本教程,接下来呢?这是一些建议:
Edit on GitHubStill need help? Join our Discord community or open a GitHub discussion