表单构造器
Actions
概述
Filament 表单可以使用 Action。Action 是可以添加到表单组件的按钮。比如你可能想要调用 API 端点来使用 AI 生成内容,或者为下拉选项框新建选项。 同时,你也可以单独渲染匿名 Action 集,而不将其附到特殊的表单组件中。
定义表单组件 Action
表单组件中的 Action 对象是 Filament/Forms/Components/Actions/Action
的实例。你必须传递一个唯一的名称到 Action 的 make()
方法,用来在 Filament 内部与其他 Action 区分开来。你可以自定义 Action 的触发按钮,甚至毫不费力地打开模态框:
use App\Actions\ResetStars;use Filament\Forms\Components\Actions\Action; Action::make('resetStars') ->icon('heroicon-m-x-mark') ->color('danger') ->requiresConfirmation() ->action(function (ResetStars $resetStars) { $resetStars(); })
将 Affix Action 添加到字段
特定字段支持 "Affix(前后缀) Action",其为输入区域前后的按钮。以下字段支持 Affix Action:
你可以传递 prefixAction
或 suffixAction
,定义一个 Affix Action:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\TextInput;use Filament\Forms\Set; TextInput::make('cost') ->prefix('€') ->suffixAction( Action::make('copyCostToPrice') ->icon('heroicon-m-clipboard') ->requiresConfirmation() ->action(function (Set $set, $state) { $set('price', $state); }) )

请注意,本例中 $set
和 $state
被注入到 action()
函数中。这是表单组件 Action utility 注入。
传入多个 Affix Action 到一个字段
你可以使用 prefixActions()
或者 suffixActions()
以数组的方式,将多个前后缀 Action 传递给一个字段。可以调用其中任意一种方法或者两者皆用,Filament 会按注册顺序进行渲染:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\TextInput; TextInput::make('cost') ->prefix('€') ->prefixActions([ Action::make('...'), Action::make('...'), Action::make('...'), ]) ->suffixActions([ Action::make('...'), Action::make('...'), ])
添加 hint action 到字段
所有的字段都支持 “Hint Action”,它渲染在字段的提示旁边。要将 Hint Action 添加到字段中,你需要将其传入到 hintAction()
中:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\TextInput;use Filament\Forms\Set; TextInput::make('cost') ->prefix('€') ->hintAction( Action::make('copyCostToPrice') ->icon('heroicon-m-clipboard') ->requiresConfirmation() ->action(function (Set $set, $state) { $set('price', $state); }) )
请注意,本例中 $set
和 $state
被注入到 action()
函数中。这是表单组件 Action utility 注入。

添加多个 Hint Action 到字段中
要将多个 Hint Action 传给一个字段y,你可以用数组的方式将它们传递给 hintActions()
。Filament 会按照 Action 注册顺序对其进行渲染:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\TextInput; TextInput::make('cost') ->prefix('€') ->hintActions([ Action::make('...'), Action::make('...'), Action::make('...'), ])
添加 Action 到自定义表单组件
如果你想在自定义的表单组件(比如,ViewField
对象或者 View
组件对象)中渲染 Action,你可以使用 registerActions()
方法:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Components\ViewField;use Filament\Forms\Set; ViewField::make('rating') ->view('filament.forms.components.range-slider') ->registerActions([ Action::make('setMaximum') ->icon('heroicon-m-star') ->action(function (Set $set) { $set('rating', 5); }), ])
请注意,本例中 $set
和 $state
被注入到 action()
函数中。这是表单组件 Action utility 注入。
现在,要在自定义组件的视图中渲染 Action,你需要调用 $getAction()
并传入你注册的 Action 名:
<div x-data="{ state: $wire.$entangle('{{ $getStatePath() }}') }"> <input x-model="state" type="range" /> {{ $getAction('setMaximum') }}</div>
添加"匿名" Action 到消息列表,而不附到组件上:
你可以使用 Actions
组件,使之在表单的任何位置中渲染一套 Action。而不必将其注册到任何特定的组件中:
use App\Actions\Star;use App\Actions\ResetStars;use Filament\Forms\Components\Actions;use Filament\Forms\Components\Actions\Action; Actions::make([ Action::make('star') ->icon('heroicon-m-star') ->requiresConfirmation() ->action(function (Star $star) { $star(); }), Action::make('resetStars') ->icon('heroicon-m-x-mark') ->color('danger') ->requiresConfirmation() ->action(function (ResetStars $resetStars) { $resetStars(); }),]),
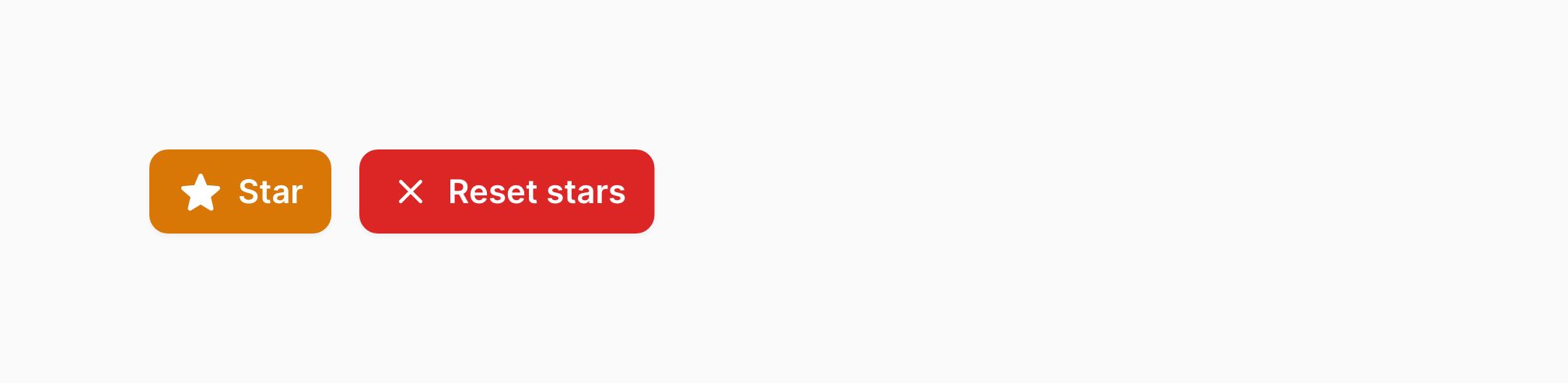
创建独立的表单组件,消费表单全部宽度
使用 fullWidth()
你可以将独立表单 Action 拉伸到占用表单的全宽:
use Filament\Forms\Components\Actions; Actions::make([ // ...])->fullWidth(),

控制独立表单 Action 的水平对齐
独立表单 Action 默认对其到表单起始位置。你可以将 Alignment::Center
或 Alignment::End
传入到 alignment()
方法,对此进行修改:
use Filament\Forms\Components\Actions;use Filament\Support\Enums\Alignment; Actions::make([ // ...])->alignment(Alignment::Center),

控制独立表单 Action 的垂直对齐
独立的表单 Action 在垂直方向上默认对齐到组件的起始位置。你可以将 Alignment::Center
或 Alignment::End
传递给 verticalAlignment()
,对此进行修改:
use Filament\Forms\Components\Actions;use Filament\Support\Enums\VerticalAlignment; Actions::make([ // ...])->verticalAlignment(VerticalAlignment::End),

表单组件 action utility 注入
如果 action 附到了表单组件,action()
函数可以直接从该表单组件中注入 utilities。比如,你可以注入 $set
和 $state
:
use Filament\Forms\Components\Actions\Action;use Filament\Forms\Set; Action::make('copyCostToPrice') ->icon('heroicon-m-clipboard') ->requiresConfirmation() ->action(function (Set $set, $state) { $set('price', $state); })
表单组件 Action 也可以访问所有通常用于该 Action 的 utilities。
Edit on GitHubStill need help? Join our Discord community or open a GitHub discussion