表单构造器 - 字段
Text input
概述
文本输入(TextInput)字段允许你使用字符串:
use Filament\Forms\Components\TextInput; TextInput::make('name')

设置 HTML input 类型
你可以使用一系列方法设置字符串类型。比如,email()
,同时也提供了相应的验证:
use Filament\Forms\Components\TextInput; TextInput::make('text') ->email() // or ->numeric() // or ->password() // or ->integer() // or ->tel() // or ->url()
你也可以使用 type()
方法,并传入一个 HTML Input 类型:
use Filament\Forms\Components\TextInput; TextInput::make('backgroundColor') ->type('color')
设置 HTML input 模式
使用 inputMode()
方法,你可以设置输入框的 inputmode
属性:
use Filament\Forms\Components\TextInput; TextInput::make('text') ->numeric() ->inputMode('decimal')
设置数值步长
使用 step()
方法,你可以设置的 Input 的 step
属性:
use Filament\Forms\Components\TextInput; TextInput::make('number') ->numeric() ->step(100)
自动补全文本
使用 autocomplete()
方法,你可以启用浏览器的自动补全:
use Filament\Forms\Components\TextInput; TextInput::make('password') ->password() ->autocomplete('new-password')
你也可以使用 autocomplete(false)
,作为 autocomplete="off"
的快捷方式:
use Filament\Forms\Components\TextInput; TextInput::make('password') ->password() ->autocomplete(false)
对于更复杂的自动补全选项,文本输入框也支持 datalists。
使用 datalist 自动补全
使用 datalist()
方法,你可以指定文本输入框的 datalist 选项:
TextInput::make('manufacturer') ->datalist([ 'BMW', 'Ford', 'Mercedes-Benz', 'Porsche', 'Toyota', 'Tesla', 'Volkswagen', ])
使用文本输入框时,Datalist 为用户提供自动补全选项。不过,这些选项纯属推荐,用户仍然在输入框中可以输入任何值。如果你想要限制用户输入一组预定义选项,请查阅 Select 字段。
自动大写文本
使用 autocapitalize()
方法,你可以允许浏览器自动大写文本:
use Filament\Forms\Components\TextInput; TextInput::make('name') ->autocapitalize('words')
字段旁边添加前后缀文本
使用 prefix()
及 suffix()
方法,你可以在文本前后添加文本:
use Filament\Forms\Components\TextInput; TextInput::make('domain') ->prefix('https://') ->suffix('.com')

使用图标作为前后缀
使用 prefixIcon
和 suffixIcon
方法,你可以在输入框前面或者后面放置图标:
use Filament\Forms\Components\TextInput; TextInput::make('domain') ->url() ->suffixIcon('heroicon-m-globe-alt')

设置前后缀图标颜色
前后缀图标默认是灰色,不过你可以使用 prefixIconColor()
和 suffixIconColor()
方法将其设为不同颜色:
use Filament\Forms\Components\TextInput; TextInput::make('domain') ->url() ->suffixIcon('heroicon-m-check-circle') ->suffixIconColor('success')
可显示的密码输入
使用 password()
时,你也可以使之可见 revealable()
,这样用户可以通过点击按钮查看他们正在输入的密码的明文:
use Filament\Forms\Components\TextInput; TextInput::make('password') ->password() ->revealable()
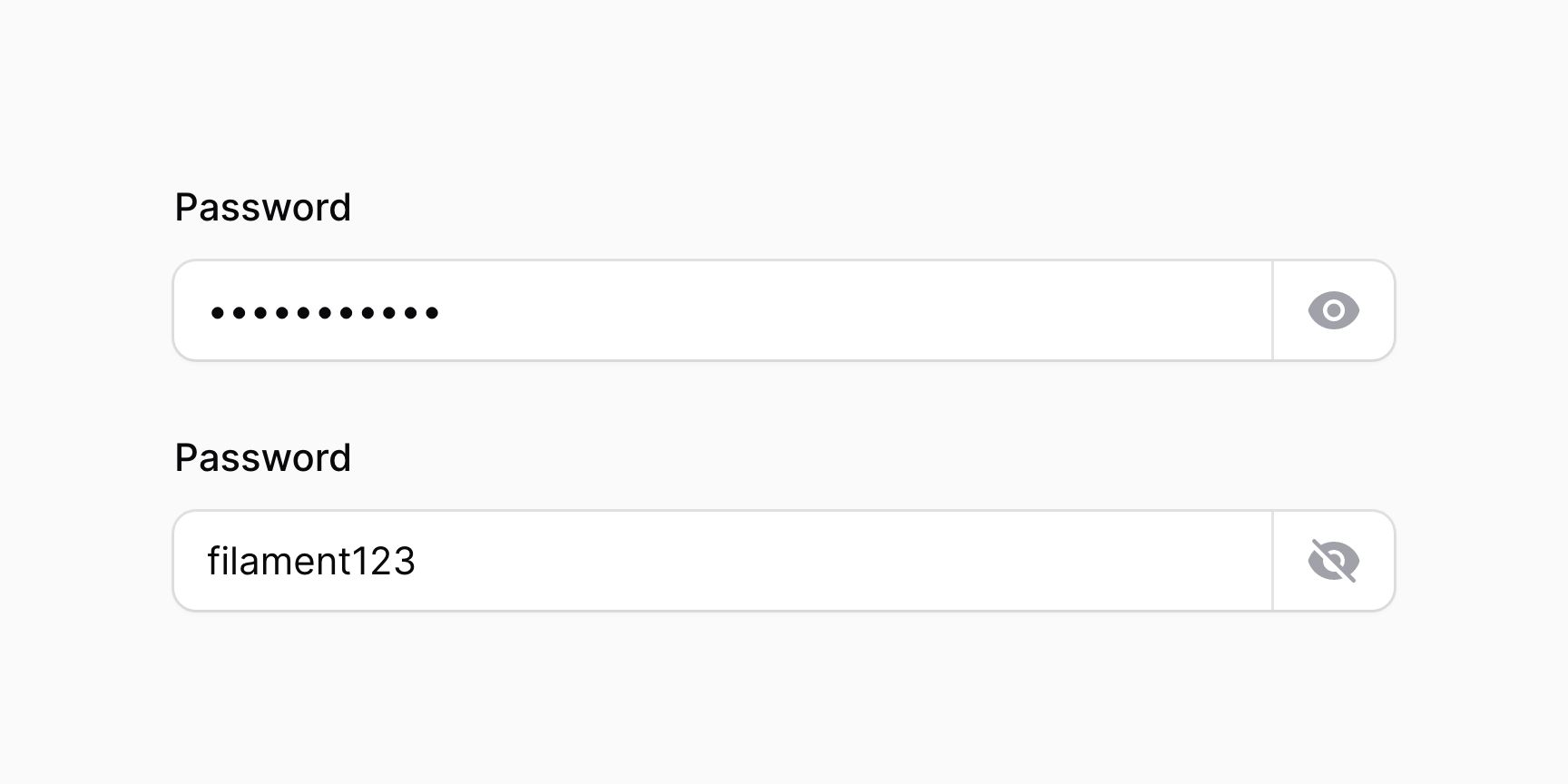
输入掩码
输入掩码(Input Masking)定义了一种输入值必须遵守的格式。
在 Filament 中,你可以使用 mask()
方法来配置 Alpine 的 mask:
use Filament\Forms\Components\TextInput; TextInput::make('birthday') ->mask('99/99/9999') ->placeholder('MM/DD/YYYY')
要使用动态掩码,请在 RawJs
对象中包裹 JavaScript:
use Filament\Forms\Components\TextInput;use Filament\Support\RawJs; TextInput::make('cardNumber') ->mask(RawJs::make(<<<'JS' $input.startsWith('34') || $input.startsWith('37') ? '9999 999999 99999' : '9999 9999 9999 9999' JS))
Alpine.js 将整个掩码值发送给服务器,因此在验证字段并保存它之前,你可能需要从状态中删除某些字符。你可以使用 stripCharacters()
方法来执行此操作,传入一个字符或一个字符数组以将其从掩码值中删除:
use Filament\Forms\Components\TextInput;use Filament\Support\RawJs; TextInput::make('amount') ->mask(RawJs::make('$money($input)')) ->stripCharacters(',') ->numeric()
使该字段只读
不要与禁用字段混为一谈,使用 readOnly()
方法,你可以让该字段变为只读:
use Filament\Forms\Components\TextInput; TextInput::make('name') ->readOnly()
相比于 disabled()
,它有一些不同之处:
- 使用
readOnly()
时,该字段仍然会在表单提交时发送给服务器。使用浏览器控制台或者通过 JavaScript,仍然可以修改它的值。可以使用dehydrated(false)
阻止该行为。 - 使用
readOnly()
时,样式不会改变,比如不会减少透明度。 - 使用
readOnly()
时,该字段仍然可以获得焦点。
文本输入验证
除了验证页面中罗列的规则之外,还有一些特别针对文本输入框的另外的规则。
长度验证
通过设置 minLength()
和 maxLength()
方法,你可以限制输入的长度。这些方法同时会对前端和后端进行验证:
use Filament\Forms\Components\TextInput; TextInput::make('name') ->minLength(2) ->maxLength(255)
通过设置 length()
,你可以指定输入框的准确长度。该方法同时添加前端和后端验证:
use Filament\Forms\Components\TextInput; TextInput::make('code') ->length(8)
Size 验证
通过设置 minValue()
和 maxValue()
方法,你可以验证数值输入框的最小和最大值:
use Filament\Forms\Components\TextInput; TextInput::make('number') ->numeric() ->minValue(1) ->maxValue(100)
电话号码验证
使用 tel()
字段时,字段值会使用 /^[+]*[(]{0,1}[0-9]{1,4}[)]{0,1}[-\s\.\/0-9]*$/
验证。
如果你想对此进行修改,你可以使用 telRegex()
方法:
use Filament\Forms\Components\TextInput; TextInput::make('phone') ->tel() ->telRegex('/^[+]*[(]{0,1}[0-9]{1,4}[)]{0,1}[-\s\.\/0-9]*$/')
此外,要全局自定义 telRegex
,请使用服务提供者:
use Filament\Forms\Components\TextInput; TextInput::configureUsing(function (TextInput $component): void { $component->telRegex('/^[+]*[(]{0,1}[0-9]{1,4}[)]{0,1}[-\s\.\/0-9]*$/');});
Edit on GitHubStill need help? Join our Discord community or open a GitHub discussion